Data Handling Entries
Data entry is a key automation problem which can be solved by using all the Best Practices that have been previously outlined. On creation of these assets, a key benefit will be that, with little adjustments, these will be portable to other projects and use cases.
Below is the example testcase which we will apply the best practices against to automate for this section:
- Launch Browser to Nopcommerce.com and validate.
- Click Register on top menu pane.
- On the Registration page, add the following data:
- Gender: Female
- First name: Testing
- Last name: 123
- Date of birth: 1/1/1990
- Email: testing@email.com
- Password: testing123
- Confirm Password: testing123
- Click Register button
- Validate Your registration completed is displayed.
Step 1: AUT Observations
- Identify the key data inputs on the page.
- Determine the user interactions that can be done to input data per scenario (text-box / drop-down)
- Determine any potential complexity.
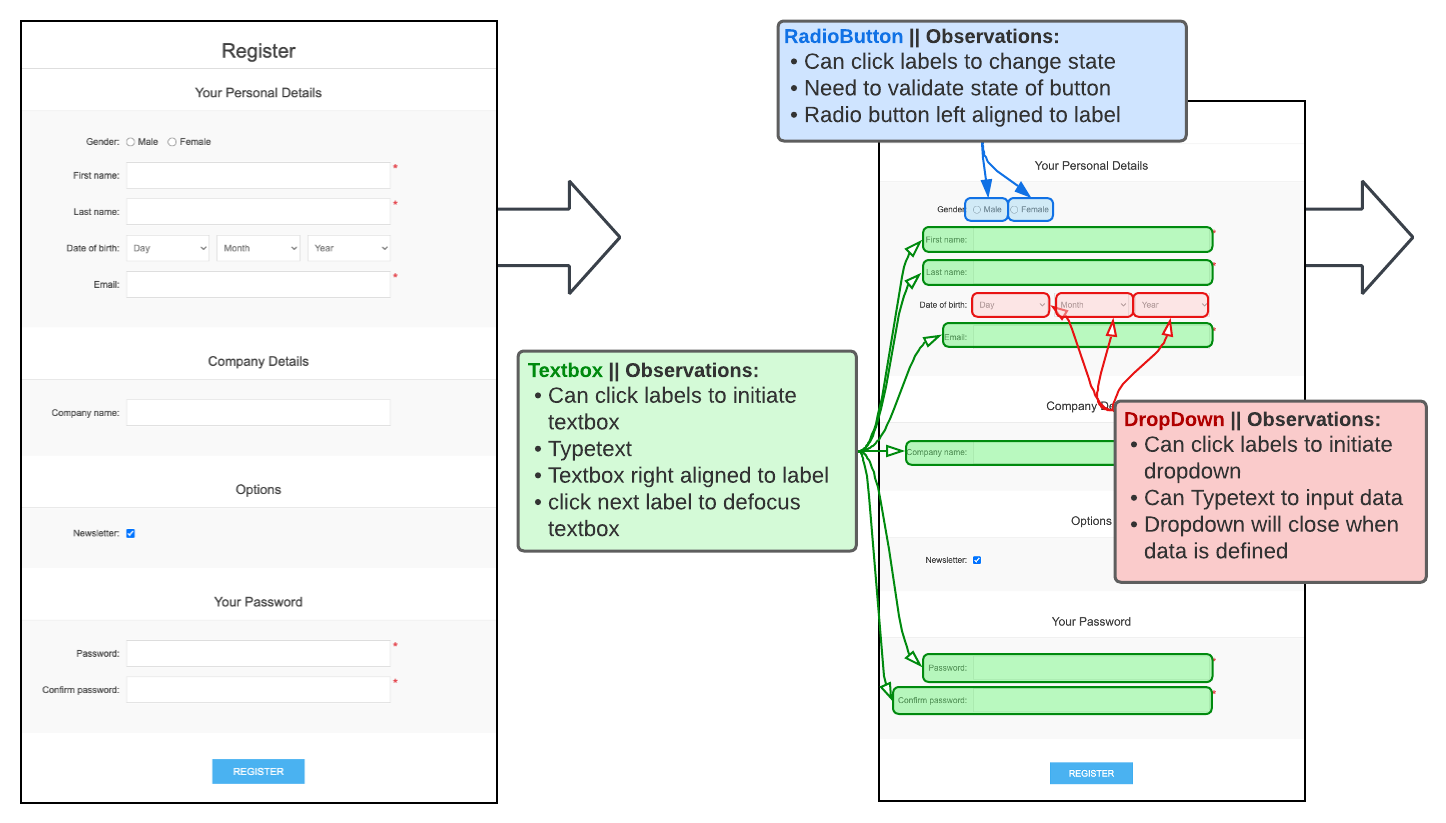
to textBox label,input
// user interactions here
end textBox
to dropDown label,input
// user interactions here
end dropDown
to radioButton label,input
// user interactions here
end radioButton
Step 2: Images Or OCR
As we can see in the screenshots and video, the user interface is heavily text driven with little to no icons. Therefore the primary automation approach would simply be OCR. However, there is one instance where we would need to capture the radio button toggle images (on/off) and had the testcase included, the checkbox image states would also be required.
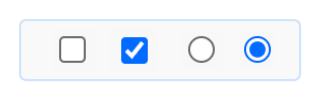
Step 3: Modular Functions
As per our best practices, and with maintenance, reusability and scalability in mind: creating 3 functions/handlers to deal with the like for like functionality will ensure we can adapt quickly if changes are applied to the form in the future.
OCR has been determined to drive the 3 types of interactions that can occur during this data entry phase. (text boxes, radio buttons and drop-downs)
Using our observations from Step 1, we now need to recreate the user interactions to scripts for our remaining actions.
TextBoxFlow: A user finds the field label, clicks on the label and types their input:
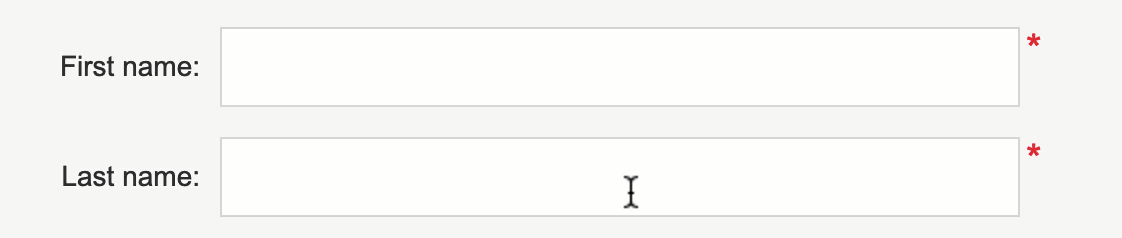
Observations:
- Clicking on the label initiates the text-box
- Animation pause between clicking label and initiating text-box
- Typing in input
Dropdown:
Flow: A user finds the dropdown label, clicks on the label and types their input:
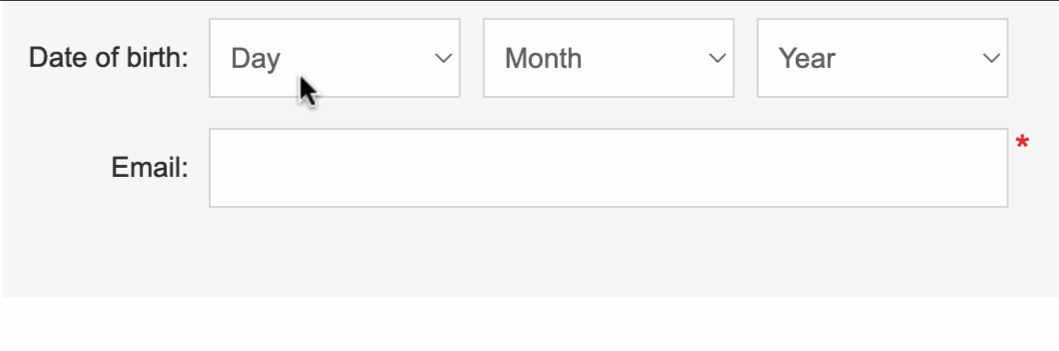
Observations:
- Can't initiate dropdown with field label
- Initiate dropdown with its initial value (day / month / year)
- Animation pause between clicking label and initiating text-box
- Typing in input
Radio Button
Flow: A user finds the radio-button category label, clicks on the option and checks the correct outcome:

Observations:
- Can't initiate radio button with field label
- Initiate dropdown with its option label value (Male / Female)
- animation pause between clicking label and initiating text-box
- validation of state (selected / not elected)
Outcome: The text-box function will be relatively simple but more importantly, it can be re-used as part of the date picker functionality. The main aspect we need to consider is the identifying of the main label for the date picker, "Date of birth", which will need to be in view before we can proceed in entering data. This reuse of existing assets is a prime example of reusability to limit effort. Resulting functions are as below. However, with the radio-button, we are not validating its resulting state is correct. Something that we will cover in the next section.
Textbox Function: | Date Picker Function: | Radio Button Function: |
---|---|---|
|
|
|
Validation of states - Offsets
In the our previous example of the radioButton function, we built a solution capable of finding the field and interacting with the element. However, our issue now is determining if the state of the radio-button is correct (on or off / true or false). To accommodate this, we need to extend our function to include a search area to find a version of the radio button icon, that corresponds to our expectation.
These search areas, are dynamically determined by the location of the text or image element we want to find and by adding or subtracting the offset based on the requirements of the validation or search. We can do this by using the imageRectangle command. In the example we subtract our expected offset from the determined location of the field.
Offsets can also be used to readText based on anchor points like images and text onscreen.
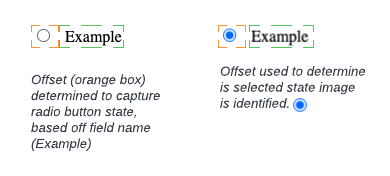
Original Radio-button Function: | Updated Radio-button Function: | |
---|---|---|
| ![]() |
|
A Summary of What You Have Learned
A fundamental best practice of automation development, is to ensure that the scripts are as modular and resuable as possible. Grouping reoccurring functionality and simplifying the code using parameters ensure that the scripts are more maintainable.
click text:"Female"
click text:"First name"
typetext "Test"
click text:"Last name"
click text:"Date of birth"
typetext "19"&tabkey&"November"&tabkey&"1987"
click text:"Email"
typetext "test@email.com"
click text:"Company name"
typetext "Test Company Name"
click text:"Password"
typetext "Password123"
click text:"Confirm password"
typetext "Password123"
radioButton ("Gender"),("Female")
textBox("First name"),("Test")
textBox("Last name"),("User")
dataPicker ("Date of birth"),("19"),("November"),("1987")
textBox("Email"),("test@email.com")
textBox("Company name"),("Test Company Name")
textBox("Password"),("Password123")
textBox("Confirm password"),("Password123")
to textBox label, input
click center of common.scrollTo(label)
wait 0.5
typetext input
wait 0.5
end textBox
to radioButton label,option,radioButtonOffset:(-20,-2,0,7)
common.scrollTo(label)
put imageRectangle(text:option)+ radioButtonOffset into optionArea
click center of optionArea
log imagefound(0,image:"radioButton/true",searchRectangle:optionArea)
end radioButton
to dataPicker label,day,month,year
common.scrollTo(label)
textBox("Day"),(day)
textBox("Month"),(month)
textBox("Year"),(year)
end dataPicker
to dropDown label, input
click center of common.scrollTo(label)
wait 0.5
typetext input,escapeKey
wait 0.3
end dropDown