Recap
We've come to the end of the Best Practices. Below, we can expand to see what the ideal suite and its scripts should be. These are key building blocks to the Direct Script or Model based approaches which should not change.
We've built the suite ensuring the key principles of: Modularisation and Reusability are followed, while ensuring the scripts reflect the user interaction to maintain Simplicity of the automation.
Eggplant Suite:
// static parameters for execution
return {
browser:{
chrome:(0,72,1920,1038),
}
}
// function to launch browser for all browsers
to launchBrowser browser,url
set universal browser to browser
typetext windowskey,"r"
wait 1
typetext browser&&url,returnkey
waitfor 20, image:"logos" // LOGO
end launchBrowser
//function to close browser for all browsers
to closeBrowser
typetext altkey,f4
wait 0.5
end closeBrowser
// function to navigate using action and validation framework
to navigate action,validation
click text:action,searchRectangle:config().browser.(universal browser)
wait 1.4 // page transition
waitfor 20, text:validation,searchRectangle:config().browser.(universal browser)
end navigate
// function to scroll to text using exact match and return location of result
to scrollTo toFind
repeat until imagefound(
waitfor:0,
text:<not preceded by (1 to 2 characters," ") , toFind, not followed by (" ", characters)>,
searchRectangle:config().browser.(universal browser)
)
mouseScroll() //
wait 0.6
if repeatindex() > 5 then logerror "Cannot find"&&toFind
end repeat
set global searchArea to ()
return foundimageinfo().imageRectangle
end scrollTo
// scroll solution
to mouseScroll
moveto the center of RemoteScreenRectangle()
ScrollWheelDown 6
wait 0.9
end mouseScroll
to textBox label, input
click center of common.scrollTo(label)
wait 0.2
typetext input
wait 0.2
end textBox
to radioButton label,option,radioButtonOffset:(-20,-2,0,7)
common.scrollTo(label)
put imageRectangle(text:option)+ radioButtonOffset into optionArea
click center of optionArea
log imagefound(0,image:"icons/radioButton/true",searchRectangle:optionArea)
end radioButton
to datePicker label,day,month,year
common.scrollTo(label)
textBox("Day"),(day)
textBox("Month"),(month)
textBox("Year"),(year)
end datePicker
to dropDown label, input
click center of common.scrollTo(label)
wait 0.3
typetext input,escapeKey
wait 0.2
end dropDown
// function that moves to and initiates dropdown
to mainMenu menu,action,validation
moveto text:menu,searchRectangle:config().browser.(universal browser)
wait 0.3
common.navigate (action),(validation)
end mainMenu
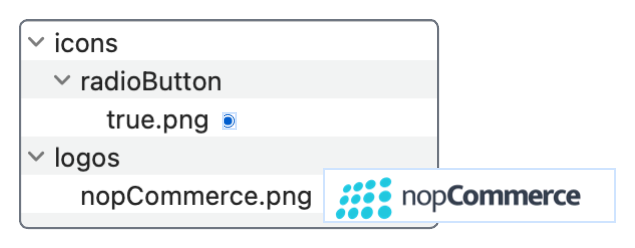
Direct Script Approach
This is first view of what the Direct Script Approach should look like. We have all 4 testcases created and ready to execute. An observation for next steps, would be looking at parameterising the first 2 testcases which share exactly the same flow but with different data.
As can be seen, the direct script approach / traditional test case management limitations are clear to see in terms of repeated effort. However, if we look at the DAI wrappers in the next section, we can see how one script can be applied to multiple instances - and will require us to do one change to the code (if required).
Script Based Testcases:
// Step: 1
common.launchBrowser ("chrome"),("https://demo.nopcommerce.com/")
// Step: 2
"custom/menu".mainmenu("Computers"),("Desktops"),("Sort by")
// Step: 3
common.navigate("Digital Storm VANQUISH 3"),("SKU: DS_VA3_PC")
// Step: 4
common.navigate ("ADD TO CART"),("The product has been added to your shopping cart")
// Step: 5
common.navigate ("Shopping cart"),("Digital Storm VANQUISH 3 Custom Performance PC")
//End
common.closeBrowser
// Step: 1
common.launchBrowser ("chrome"),("https://demo.nopcommerce.com/")
// Step: 2
"custom/menu".mainMenu("Apparel"),("Clothing"),("Sort by")
// Step: 3
common.navigate("Levi's 511 Jeans"),("SKU: LV_511_JN")
// Step: 4
common.navigate ("ADD TO CART"),("The product has been added to your shopping cart")
// Step: 5
common.navigate ("Shopping cart"),("Levi's 511 Jeans")
//End
common.closeBrowser
// Step: 1
common.launchBrowser ("chrome"),("https://demo.nopcommerce.com/")
// Step: 2
common.navigate("Books"),("Sort by")
// Step: 3
common.navigate("Pride and Prejudice"),("SKU: PRIDE_PRJ")
// Step: 4
common.navigate ("ADD TO CART"),("The product has been added to your shopping cart")
// Step: 5
common.navigate ("Shopping cart"),("Pride and Prejudice")
//End
common.closeBrowser
// Step: 1
common.launchBrowser ("chrome"),("https://demo.nopcommerce.com/")
// Step: 2
common.navigate("Register"),("Your Personal Details")
// Textstyle for data entry to ensure accuracy.
set the textStyle to (contrast:"On", contrastColor:"#737573", contrastTolerance:"99")
// Step: 3
"custom/data entry".radioButton ("Gender"),("Female")
"custom/data entry".textBox("First name"),("Testing")
"custom/data entry".textBox("Last name"),("123")
"custom/data entry".datePicker ("Date of birth"),("1"),("January"),("1990")
"custom/data entry".textBox("Email"),("testing@email.com")
"custom/data entry".textBox("Password"),("testing123")
"custom/data entry".textBox("Confirm password"),("testing123")
set the textStyle to "Default"
Step: 4 & 5
common.navigate("REGISTER"),("Your registration completed")
// End
common.closeBrowser
Model Based Approach
Below is our Model representation of our 4 testcases. Here we can see that through aggregation of the test steps and abstraction of the item catalog and item pages, we are left with a clear visual understanding of what our reoccurring test flows are.
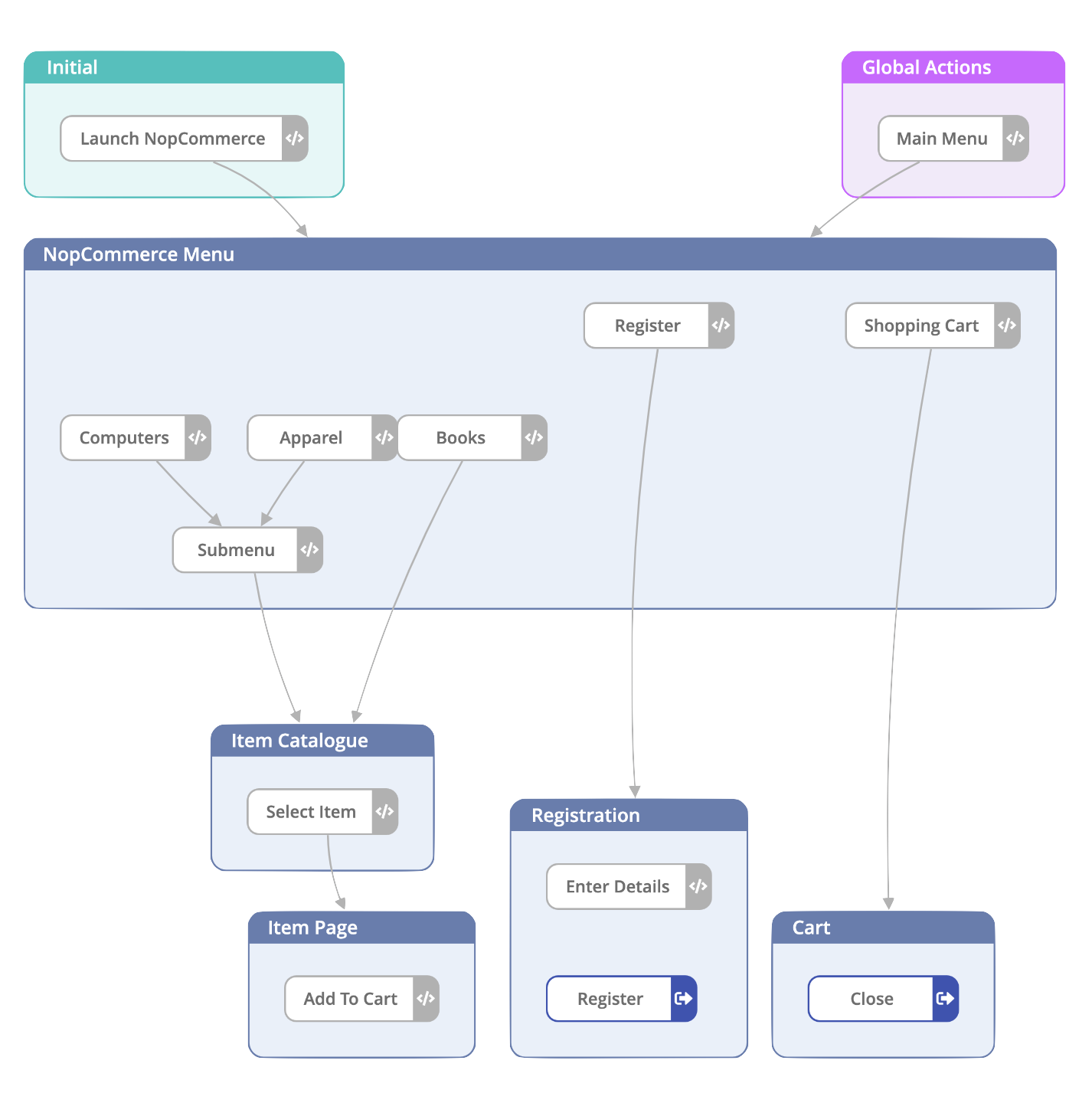
As we have abstracted out the item catalog and item pages (not modelled the specific pages and generalised the flow), we need to consider parameterising the journeys within DAI using State level variables eg. our submenu flow. The variable submenu will be provided by DAI based on the options present in the UI.
to computers submenu
"custom/menu".mainmenu("Computers"),(submenu),("Sort by")
end computers
DAI Wrappers:
to launchNopcommerce
common.launchBrowser ("chrome"),("https://demo.nopcommerce.com/")
end launchNopcommerce
to computers submenu
"custom/menu".mainmenu("Computers"),(submenu),("Sort by")
end computers
to apparel submenu
"custom/menu".mainmenu("Apparel"),(submenu),("Sort by")
end apparel
to books
common.navigate ("Books"),("Sort by")
end books
to register
common.navigate("Register"),("Your Personal Details")
end register
to shoppingCart item
common.navigate ("Shopping cart"),(item)
end shoppingCart
to close
common.closeBrowser
end close
to selectItem item, sku
common.navigate(item),(sku)
end selectItem
to addToCart
common.navigate ("ADD TO CART"),("The product has been added to your shopping cart")
end addToCart
to enterDetails
// Textstyle for data entry to ensure accuracy.
set the textStyle to (contrast:"On", contrastColor:"#737573", contrastTolerance:"99")
// Step: 3
"custom/data entry".radioButton ("Gender"),("Female")
"custom/data entry".textBox("First name"),("Testing")
"custom/data entry".textBox("Last name"),("123")
"custom/data entry".datePicker ("Date of birth"),("1"),("January"),("1990")
"custom/data entry".textBox("Email"),("testing@email.com")
"custom/data entry".textBox("Password"),("testing123")
"custom/data entry".textBox("Confirm password"),("testing123")
set the textStyle to "Default"
end enterDetails
to register
common.navigate("REGISTER"),("Your registration completed")
end register