Foreign Translations
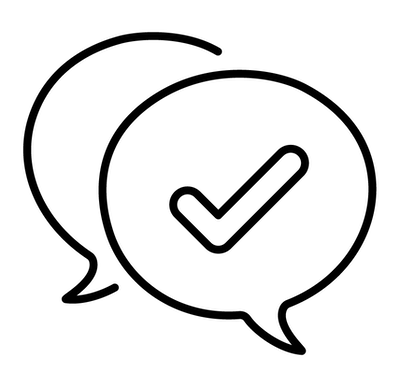
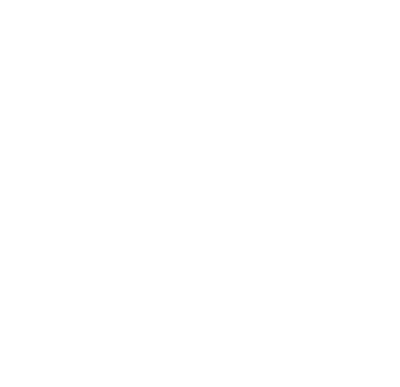
The following translations from other languages may help you to get oriented in the land of SenseTalk.
%
The %
symbol is used in many languages as a 'modulo' operator, and is most commonly used to test whether a number is even or odd, or is evenly divisible by some number. SenseTalk has mod
and rem
operators for the general case. The more common divisibility tests are written like this:
if x is an even number then
…if x is divisible by 10 then
…if x is a multiple of 25 then
…
For more information, see Is a Multiple Of, Is Divisible By Operators.
=, ==, !=
Many languages use the =
symbol for assignment and ==
to test for equality. In SenseTalk =
(or the words is
, are
, equals
, is equal to
, etc.) means equals and the symbols ==
and !=
are not used. The put
and set
commands are used for assignment. For more information, see Comparison Operators and Storing into Containers.
++, --, +=, -=
These symbols are used in many languages for incrementing or decrementing a variable, or adding or subtracting an amount from a variable. In SenseTalk, the add
and subtract
commands are used:
add 1 to x -- increment
subtract 1 from x -- decrement
add foo to x -- add an amount
subtract foo from x -- subtract an amount
For more information, see Arithmetic Commands.
nil, null
In other languages these terms are used to indicate something that does not have an assigned value. In nearly all cases, SenseTalk uses empty
for this purpose. The fact that empty is an empty string (the same as ""
) may seem odd, but in practice it works very well (if this really bothers you, perhaps it will help to think of a variable as a box: when the box has nothing in it, it is empty; but the box is still there). For the rare cases when it is important to distinguish between empty
and the complete absence of a value (primarily when working with databases, or converting to or from JSON or XML-RPC objects), SenseTalk also has the value missing value
(and its synonyms, nil
and null
). The rarely-used term nullChar
represents the null character (the same as numToChar(0)
).
array, matrix, list
A "list" in SenseTalk is a variable-length sequential collection which may contain any mixture of values, including other lists. Use the terms item
or items
to refer to items in the list. SenseTalk lists use a sparse array implementation, so storing a value into item 1000000 of a list uses no more memory than storing into item 100.
hash, hash table, dictionary, associative array
A "property list" or "object" in SenseTalk is a collection of keys and values.
regular expressions, regex
SenseTalk calls these "patterns" and includes an English-like pattern language for defining them.
eval, exec
The SenseTalk value
function evaluates a string expression and returns its value. The do
command executes a string containing lines of code. The shell
command and function executes code in the shell environment.
for, while, do
All repeat loops in SenseTalk start with a line beginning with the word repeat
, and end with end repeat
on its own line. There are many varieties of repeat loops, covering all of the usual cases, plus a few unique ones like repeat for [a duration]
(e.g., repeat for 15 seconds
) and time-based (e.g., repeat until "5:00 PM"
).
switch / case
The SenseTalk equivalent of a case statement in other languages is called a "multi-case if" statement, which is a variation on if
that breaks the conditional into two parts separated by "…".
if color is …
… "red" then doSomethingRed
… "green" then becomeGreen
… "blue" then singTheBlues
else put "I don't know that color"
end if
break
To exit from a repeat loop, SenseTalk uses the command exit repeat
. Branches of multicase if statements do not automatically fall through to execute the next branch, as they do in some languages, so no equivalent to break is needed. To force execution to continue with the next case, use the command execute the next case
.
continue
To jump back to the top of a repeat loop and continue with the next iteration (if any), use the command next repeat
.
0, 1
Numbers are not valid boolean values in SenseTalk. Use true
, false
, yes
, no
, on
, or off
(including their string representations, "true"
, "false"
, etc.). Empty
is also valid (treated as false) in a conditional expression.
&&, ||
The logical operators in SenseTalk are and
and or
(&
and &&
are the concatenate and concatenate-with-space operators). And
and or
are not "short-circuit" operators (both expressions are fully evaluated before the operators are applied). If short-circuit behavior is important, you can use and if
and or if
instead.
&, |, ^, ~, <<, >>
The bitwise operators in SenseTalk are called bitAnd
, bitOr
, bitXor
, bitNot
, and bitShift
.
/**/
Block comments in SenseTalk use the symbols (*
and *)
. Block comments can be nested, which is useful when commenting out blocks of code that contain other block comments.
?
SenseTalk’s equivalent of the so-called “ternary operator” is the ‘(if
… then
… else
…)’ operator or “selector expression” and can be used for values (to select a variable being assigned to) as well as values. For more information, see the (If... Then.... Else...) Operator.
!
The logical “not” operator in SenseTalk is not
.
self, this
SenseTalk uses the terms me
or my
to refer to the object whose script is executing. For more information, see Using “Me” and “My” to Access an Object’s Own Properties.