SenseTalk Travel Guide
A Guidebook for Experienced Coders
SenseTalk is an "intuitive scripting language" — which is great for people new to programming, but may be disorienting (and at times even counter-intuitive) for people — perhaps you — who already know another language.
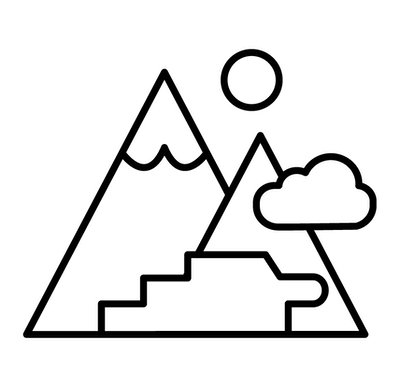
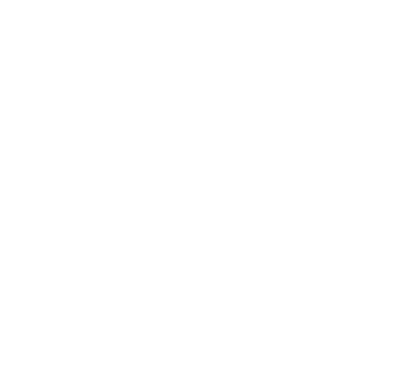
The Expedition: To cross the borders into this new territory and master the language well enough to be able to converse comfortably with the natives.
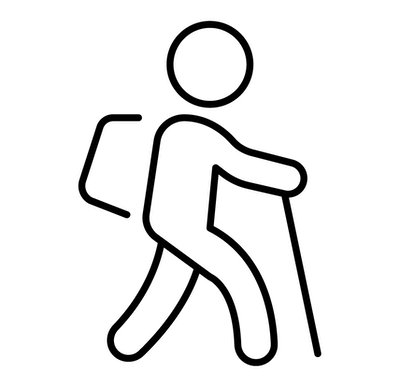
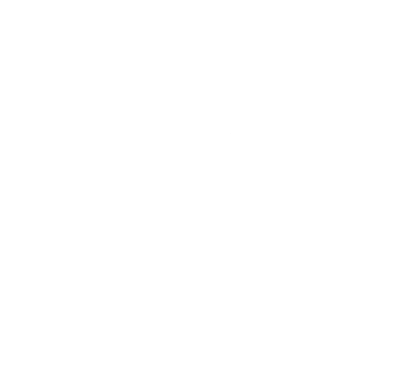
The Explorer: You, an experienced traveler in other languages, well equipped with the latest coding skills and tools. You expect to quickly be speaking the lingo, but troubles await…
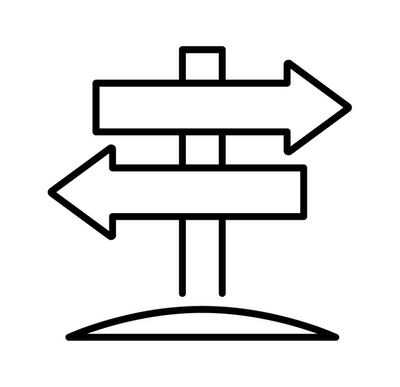
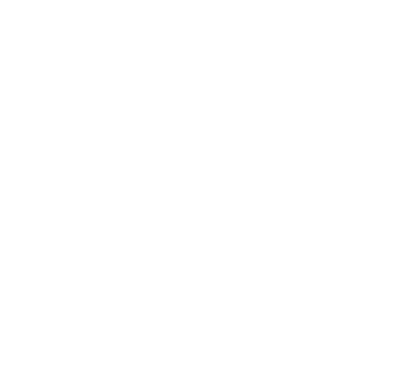
The Challenge: SenseTalk presents new and unfamiliar terrain. Familiar operations are accomplished in unfamiliar ways. Your mastery of programming concepts is a tremendous asset, but some of the specific skills and techniques you've learned with other languages may actually hold you back. Some of your ingrained assumptions could mislead you down the wrong path toward a slippery slope that will tumble you into the ravine of frustration.
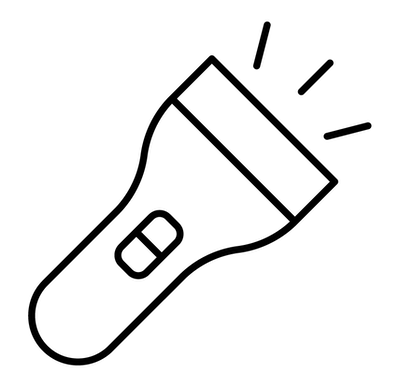
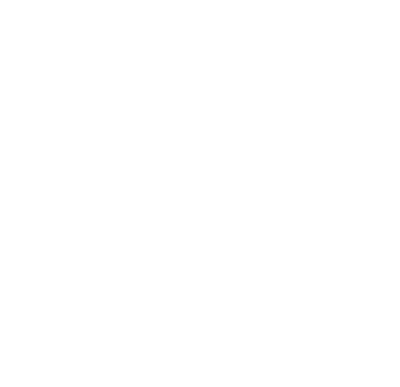
Emergency Supplies: Our aim is to get you up to speed quickly, and point out some of the pitfalls and peculiarities that may get in your way. We'll try to guide you safely along the path to the summit.
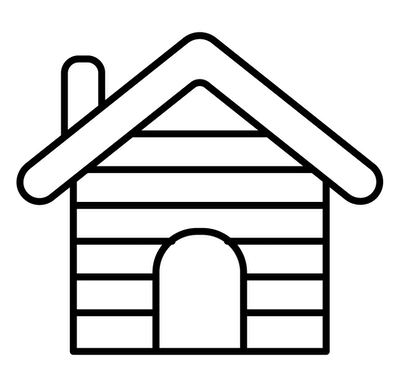
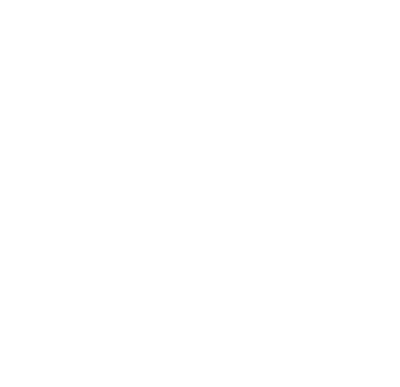
Base Camp: We provide hints here to point you in the right direction. For the complete map of the territory, be sure to refer to the documentation.
Things Are Different Here
Be alert for the unexpected, and come back to this guide whenever you need to.
Lay of the Land
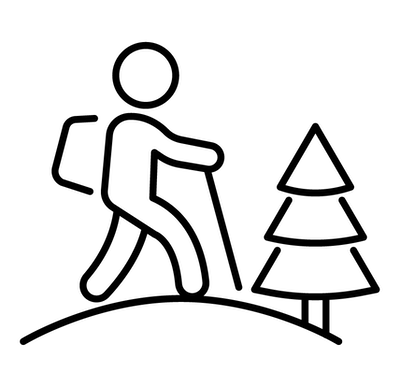
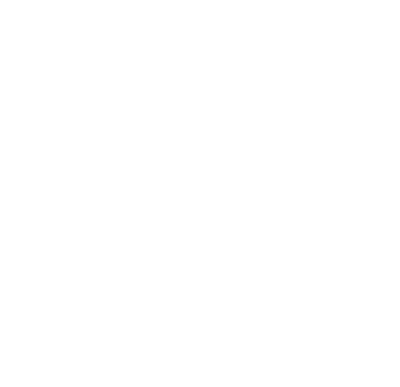
The natives here speak a strange dialect of English. That should be helpful since you may speak English conversationally at home … but never when you're traveling in the lands of code! So it may feel like a foreign language in these parts. Learn the basics of the SenseTalk dialect, though, and you'll get along just fine — for example, statements are all imperative commands that begin with a verb, each on a separate line:
sort myList
add 1 to total
delete line 3 of text
Keep in mind that SenseTalk is a People Oriented Programming language. The emphasis is on making it easy for ordinary people to accomplish a task, but what's intuitive for "people" may at times feel counterintuitive to you as an experienced coder. So relax your assumptions and shift your thinking to see it from the "people" perspective and you'll have an easier time getting along with the locals.
Things mean what they do in the world. A percent sign means percent. Quoted literal strings are exactly as they appear: "\n"
is a two-character string containing a backslash and an 'n', not a newline (but see below, if you really must have escape sequences).
String Literals Are Actually Literal
The string "ABC\n123" has 8 characters, and characters 4 and 5 are "\" and "n". If you want a string with "ABC" on one line and "123" on the next, that can be done in several ways:
String concatenation (&):
set text to "ABC" & return & "123"
String concatenation with embedded space (&&):
set text to "ABC" && "123" -- (ok, there's no return in this example)
Use "!" before a literal to evaluate embedded expressions in double square brackets:
set text to !"ABC[[return]]123"
Use block quoted string literals:
set text to {{
ABC
123
}}